In React, the concepts of “map” and “key” are essential for efficiently rendering lists of elements. Here’s a brief introduction to each:
Map Function in React
The map
function is a JavaScript array method that creates a new array populated with the results of calling a provided function on every element in the calling array. In React, it’s commonly used to transform an array of data into an array of JSX elements.
Example
import React, { StrictMode } from "react";
import { createRoot } from "react-dom/client";
const numbers = [1, 2, 3, 4, 5];
const listItems = numbers.map((number) =>
<li>{number}</li>
);
const root = createRoot(document.getElementById("root"));
root.render(
<StrictMode>
<ul>{listItems}</ul>
</StrictMode>
);
Result:
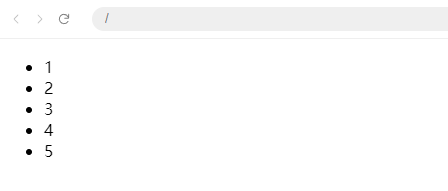
In this example, numbers.map()
creates a new array of <li>
elements, each containing a number from the numbers
array.
Keys in React
A “key” is a special string attribute you need to include when creating lists of elements in React. Keys help React identify which items have changed, been added, or removed. This improves the efficiency of updates and helps maintain the identity of each component.
import React, { StrictMode } from "react";
import { createRoot } from "react-dom/client";
const numbers = [1, 2, 3, 4, 5];
const listItems = numbers.map((number) =>
<li key={number.toString()}>{number}</li>
);
const root = createRoot(document.getElementById("root"));
root.render(
<StrictMode>
<ul>{listItems}</ul>
</StrictMode>
);
In this example, each <li>
element is given a key
attribute set to the corresponding number’s string representation. This key should be a unique identifier for each element in the array.
.